Mampac
New member
- Joined
- Nov 20, 2019
- Messages
- 49
Hi there,
I'm doing a project for my programming school which concerns ray-casting to render images for a game.
The game is defined on a 2D-grid map, where the squares are either empty spaces, where the player can freely move, or solid walls.
Therefore the Player is always standing inside an empty square. They are looking in a direction defined by a direction vector, whose X and Y, called dirX and dirY, are in range [-1, 1].
The Player, then, emits a number of rays from its position to look to the left and to the right of their direction vector.
The program has to understand when the ray hits a wall. Since adding a constant value is inefficient (the ray can "skip" the wall; thus an infinitely small constant has to be added, which is impossible), there's an algorithm to add a value which will jump exactly on square sides:
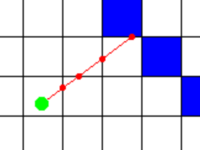
The material I was provided with proposes the following algorithm:
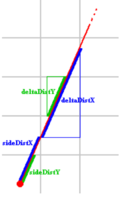
Where deltaDistY is the distance from one horizontal, y-side to another and deltaDistX is the distance from one vertical, x-side to another (sideDistX, sideDistY are the initial distance from the player's location, but it's not the matter in this case).
The author states that geometrically, the deltas, using Pythagoras, are equal to:
[MATH]deltaDistX = \sqrt{1 + {\dfrac{rayDirY^2}{rayDirX^2}}}[/MATH][MATH]deltaDistY = \sqrt{1 + {\dfrac{rayDirX^2}{rayDirY^2}}}[/MATH]
What I do see is that the deltas are essentially the hypotenuses of the triangles; one of their sides is always 1 (the side of a square), but how is the other leg found? Why are the coordinates squared and divided by each other? This doesn't fit in any formulae I know about vectors (say norm of a vector, distance between points etc.) The author simplifies the formula further, by stating that
[MATH]deltaDistX = |{\dfrac{|v|}{rayDirX}}|[/MATH][MATH]deltaDistY = |{\dfrac{|v|}{rayDirY}}|[/MATH]
where [MATH]v[/MATH] is the length of the ray's direction vector (which depends on the player's direction vector, which is a variable), and is equal to [MATH]\sqrt{rayDirX^2 + rayDirY^2}[/MATH].
The author didn't go into math deeply, because they were rather focused on the coding aspect of the guide, so I'm stuck at this point and can't proceed. I'm taking Linear Algebra class this semester; it surely did aid me in grasping the material but I, including a couple of my friends I asked for help, failed to recall such formulae. Can anyone help me?
I'm doing a project for my programming school which concerns ray-casting to render images for a game.
The game is defined on a 2D-grid map, where the squares are either empty spaces, where the player can freely move, or solid walls.
Therefore the Player is always standing inside an empty square. They are looking in a direction defined by a direction vector, whose X and Y, called dirX and dirY, are in range [-1, 1].
The Player, then, emits a number of rays from its position to look to the left and to the right of their direction vector.
The program has to understand when the ray hits a wall. Since adding a constant value is inefficient (the ray can "skip" the wall; thus an infinitely small constant has to be added, which is impossible), there's an algorithm to add a value which will jump exactly on square sides:
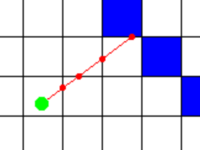
The material I was provided with proposes the following algorithm:
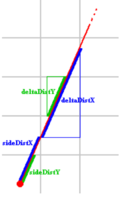
Where deltaDistY is the distance from one horizontal, y-side to another and deltaDistX is the distance from one vertical, x-side to another (sideDistX, sideDistY are the initial distance from the player's location, but it's not the matter in this case).
The author states that geometrically, the deltas, using Pythagoras, are equal to:
[MATH]deltaDistX = \sqrt{1 + {\dfrac{rayDirY^2}{rayDirX^2}}}[/MATH][MATH]deltaDistY = \sqrt{1 + {\dfrac{rayDirX^2}{rayDirY^2}}}[/MATH]
What I do see is that the deltas are essentially the hypotenuses of the triangles; one of their sides is always 1 (the side of a square), but how is the other leg found? Why are the coordinates squared and divided by each other? This doesn't fit in any formulae I know about vectors (say norm of a vector, distance between points etc.) The author simplifies the formula further, by stating that
[MATH]deltaDistX = |{\dfrac{|v|}{rayDirX}}|[/MATH][MATH]deltaDistY = |{\dfrac{|v|}{rayDirY}}|[/MATH]
where [MATH]v[/MATH] is the length of the ray's direction vector (which depends on the player's direction vector, which is a variable), and is equal to [MATH]\sqrt{rayDirX^2 + rayDirY^2}[/MATH].
The author didn't go into math deeply, because they were rather focused on the coding aspect of the guide, so I'm stuck at this point and can't proceed. I'm taking Linear Algebra class this semester; it surely did aid me in grasping the material but I, including a couple of my friends I asked for help, failed to recall such formulae. Can anyone help me?